JavaScript is a programming language created in 1995 for the Worldwide Web – and now for a lot of websites. It helps us know how to start with javascript and create web apps.
If done correctly, it can be a user-friendly space, interactive, and easy to use.
JavaScript became versatile over the years. You can now use it for the back-end development using NodeJS and build iOS and android apps using ReactNative.
You van even build desktop apps across platforms using Electron.
Now that we know what JavaScript is, let’s start with the basic syntax of JavaScript.
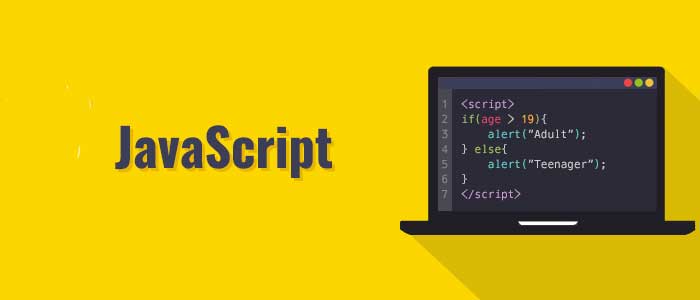
How To Start Learning With JavaScript?
Below are the beginners topics or things that you should learn before learning a actual course. Because, while knowing these basics terms of JavaScript.
You will have better understanding of advanced topics that comes in JavaScript Tutorials.
Comments
These are used to organise the code as well as letting the person looking at the code know what is happening. There are two types of comments – single-line and multi-line comments.
Single-line comments
As the name suggests – it is on a single line which can be written using 2 forward slashes.
//This is where it starts.
Multi-line comments
Similarly, it is a comment written on multiple lines using forward slash and asterisk at both ends.
/* this can
also be where
it starts*/
Variables
Variables are where we store the data. Keywords like var, let, and const are used to declare the variables.
JavaScript has a way where every word starts with a lower-case symbol. It automatically becomes upper-case when the next word is entered.
var variableName;
let variableName;
const variableName;
Because JavaScript is case sensitive VariableName is not the same as variableName.
You can use var, let and const to declare variables, but e=what is the difference??
With var and let there is no fixed value. But with const you cannot change the value once fixed.
//this is right
var flower = “sunflower”;
flower = “rose”;
//this is right
let flower = “sunflower”;
flower = “rose”;
//this is NOT right
const flower = “sunflower”;
flower = “rose”;
From the above examples we can see which is wrong and which is right. The var and let statements are right whereas, the const statements aren’t.
It is because the values can be changed for var and let, but for const it can be only “sunflower” and nothing else.
So, what is difference between var and let?
var can be accessed in a function just once while both let and const can only be accessed in a block. A block is anything within a parenthesis.
for(let i=1;i<=0;i++)
{
var animal=”horse”;
country=”Italy”;
} // here the let has declared I as the variable and it won’t interfere with anything inside the “{}” parenthesis while var can declare any variable inside the “for” function.
There are Literals, which are fixed data. You cant change it i.e. the user has no control over the literals unlike with variables.
45
“Welcome to JavaScript”
Let’s Understand How JavaScript Starts?
While learning the above terms, you become familiar with JavaScript Programming languages, and it will help you understand before you learn about operators, assignments etc.
Operators
These are what we use in for mathematical calculations or to assign values.
variableValue = 45;
Here, the operator is “=”. Similarly, if you want to add you use “+”. If you want to subtract use “-“. If you want to multiply use “*”.
value1 = 4+5;
value2 = 4*5;
value3 = 5-4;
If you want to divide you use “/” and if you want to find a remainder then use”%”.
value4 = 6/2;
value5 = 5%2;
Assignments
We saw earlier how we can assign values to variables, but we can also assign a variable to another variable.
var a = 4;
b = a; // this means both a and b have the value 4 assigned to them.
You can add variables to assign a new variable.
var a = “welcome”;
a+= “home”; //initially the value of a was ‘welcome’ but after the second operation it is ‘welcome home’
You can do this with numerical values as well in a similar way.
var a=1;
a+= 1; // value of a is 2, can even write this operation as a++
a*=4;// value of a is 8
a/=2; // value of a is 4
a%=3;//value of a is 1
Data Types
There are different types of data types in JavaScript. Namely –
String
These variables are the type to store data in the form of text. To show that the text is a value, you must enclose it in single quote marks.
let a=’charlie’;
Number
These variables are the type to store data in the form of integers. There is no need of single quote marks to assign it to a variable.
let a =7;
Boolean
These variables have only two values – true or false. There is also no need for single quote marks here as well.
let a=true;
Array
In this type of variable, you are storing multiple values in a single variable. It is enclosed in “[]” parenthesis, and the values inside have the quote marks if they are of the string type.
let a=[4,’harsh’,’charlie’,7];
//you refer to the elements in the array as a[0],a[1], etc.
Conditionals
They are a form of code to see if the parameters set to it return true or not. The most popular one being the if…else conditional.
let a=2;
if(a%2==0)
{
alert(“even”);
}
else
{
alert(“odd”);
}
In the above code, the program first calculates the remainder of a%2 and then if it is 0. If it is then the program will go to the first block and print “even”.
Supposing it fails, the program will skip the first block and go the second block and print “odd”.
Functions
They are the already existing code in the program which you can use continuously, without having to define it again and again.
If you see anything that looks like it could be the name of a variable but “()” at the end – then it’s a function.
We have already used some already like alert. These functions help us code faster with the already existing basic functions. We can use these to save time and focus on the more difficult problems.
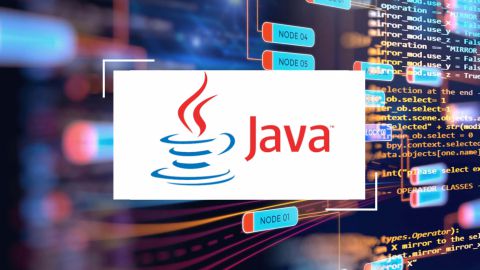
Conclusion
If you have read through the article, then you should have a grasp at the basics of JavaScript.
By learning the basics you have now built a strong foundation, should you go on to learn the advanced techniques.
Read: How To Start Coding with Javascript
To be honest, I feel like you should learn even the advanced techniques of JavaScript. This will help you thorough understand from how to start with javascript to advanced level of JavaScript.
Since, it has such a high scope for your personal development, you should. Also, there are numerous job opportunities for people with coding skills.
I hope you continue this path and develop your skills as you go.
Good luck!!